2D Elasticity problem#
The elasticity problem illustrates how to use vector unknown in XLiFE++:
For homogeneous isotropic material:
The variational formulation in
This is implemented as follows:
#include "xlife++.h"
using namespace xlifepp;
// data function
Vector<Real> f(const Point& P, Parameters& pa = defaultParameters)
{ Vector<Real> F(2, 0.); F(2)=-0.005; return F;}
int main(int argc, char** argv)
{
init(argc, argv, _lang=en); // mandatory initialization of xlifepp
// mesh rectangle
Rectangle rect(_center=Point(0., 0.), _xlength=20, _ylength=2, _nnodes=Numbers(50, 5), _domain_name="Omega", _side_names=Strings("", "", "", "Gamma"));
Mesh mesh2d(rect, _shape=triangle, _generator=gmsh);
Domain omega=mesh2d.domain("Omega"), Gamma=mesh2d.domain("Gamma");
// create P1 Lagrange interpolation
Space V(_domain=omega, _interpolation=P1, _name="V");
Unknown u(V, _name="u", _dim=2); TestFunction v(u, _name="v");
// create bilinear form and linear form
Real lambda=167.06, mu=56,67, omg2=0, rho=7.86;
BilinearForm auv = lambda*intg(omega, div(u)*div(v)) + 2*mu*intg(omega, epsilon(u) % epsilon(v)) - omg2*intg(omega, u|v);
LinearForm fv=intg(omega, f|v);
EssentialConditions ecs= (u|Gamma=0);
TermMatrix A(auv, ecs, _name="A");
TermVector B(fv, _name="B");
//solve linear system AX=B using direct method
TermVector U=directSolve(A, B);
thePrintStream<<U;
saveToFile("U", U, _format=vtu);
// create the deformation of the mesh
for (number_t i=0;i<mesh2d.nbOfNodes();i++)
mesh2d.nodes[i] += U.evaluate(mesh2d.nodes[i]).value<std::vector<Real> >();
saveToFile("Ud", mesh2d, _format=msh);
return 0;
}
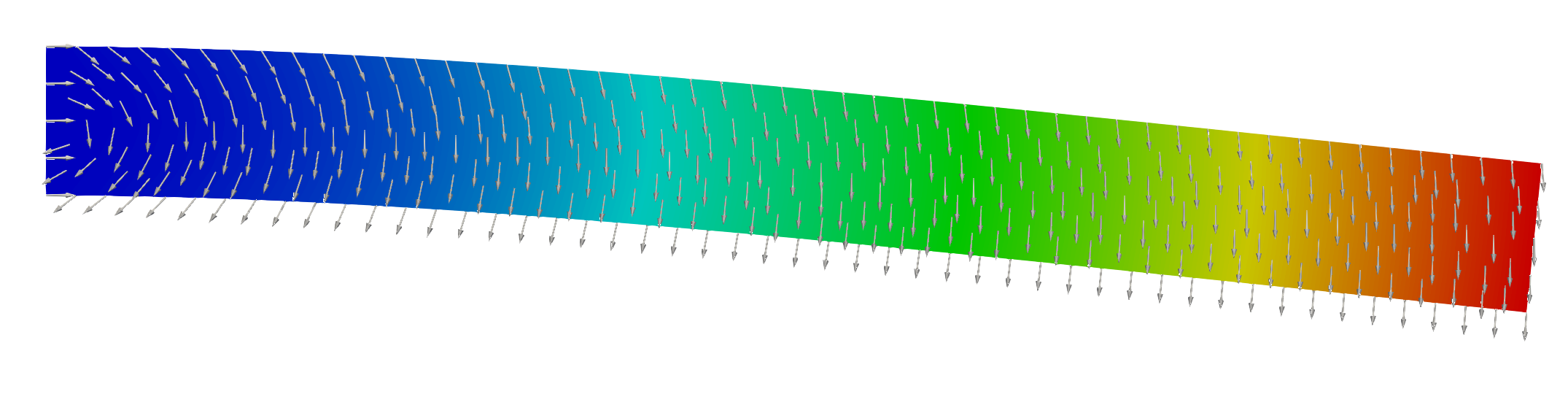
Fig. 31 Displacement and modulus of the solution of the elasticity 2D problem#